Azure DevOps has a list of data columns that is not displayed on detail sheet. Try to figure out the column exists before adding a new column,
We would like to add a new column, “Acceptance Criteria”.
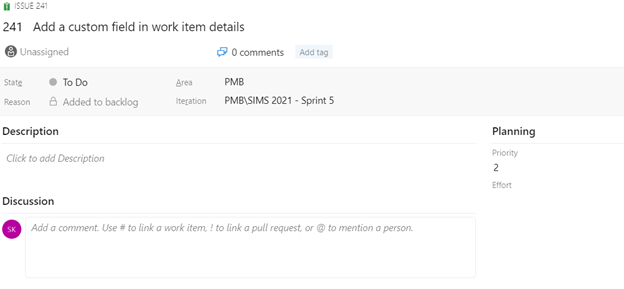
You can click on three dots (…) in the upper right corner of task detail and select Customize.
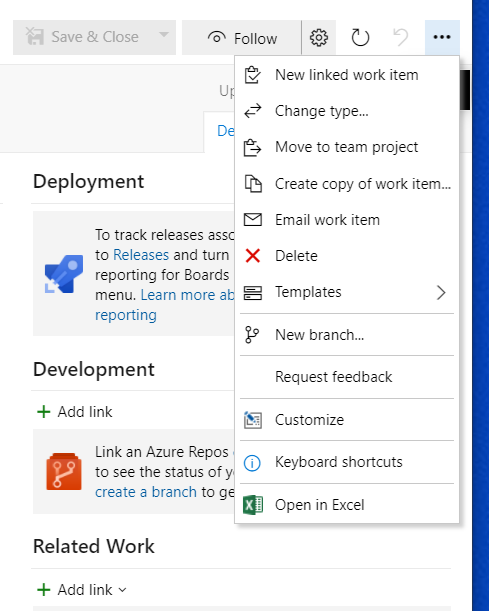
This will take you to the Process section of Organization settings page. Alternatively, you can cick on Organization Settings -> Boards -> Process.
Before adding new column;
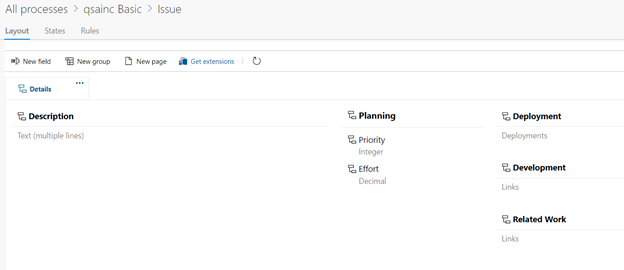
Click on “New field”. This will pop up a new window;
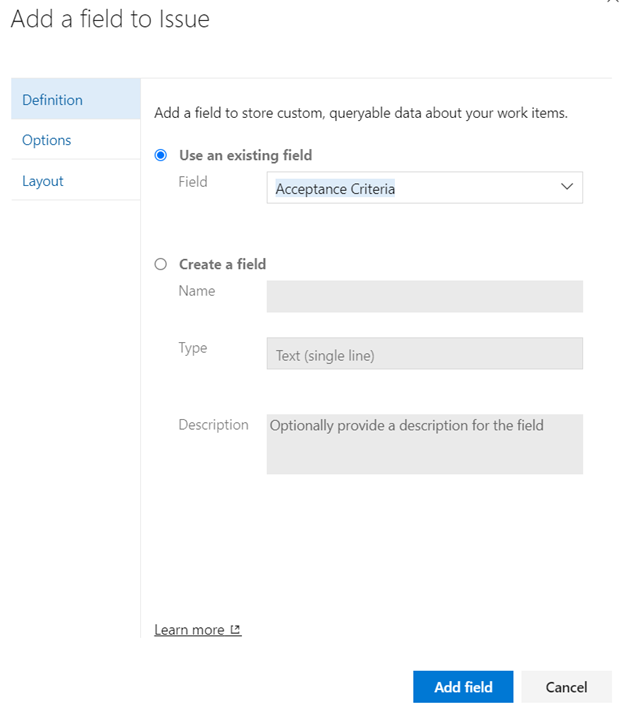
Since the field is already created so I am using “Use an existing field” option. You can create a new field if it does not exist.
If you want to make it as required field, click on “Options”.
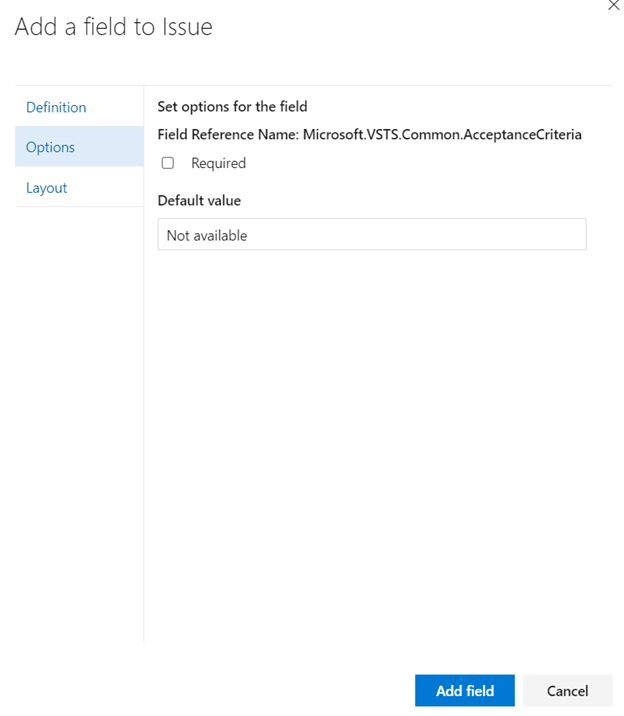
I just left Layout as default.
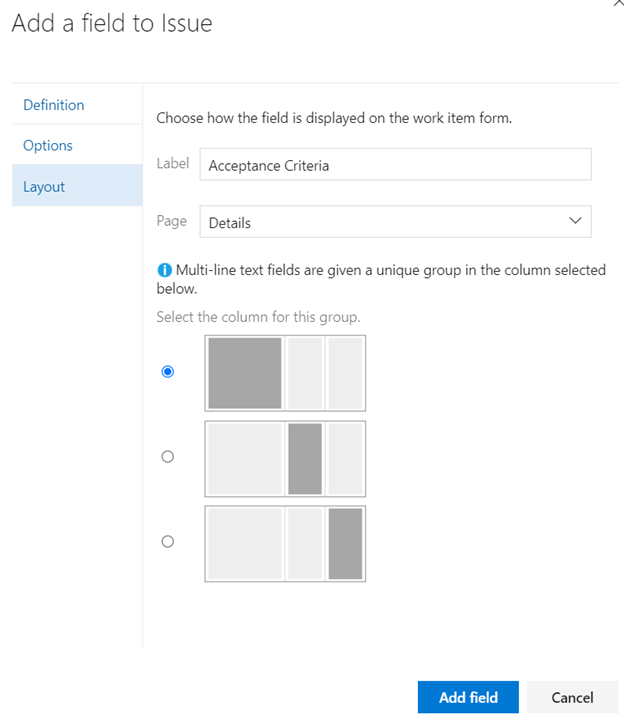
Click on “Add field” button.
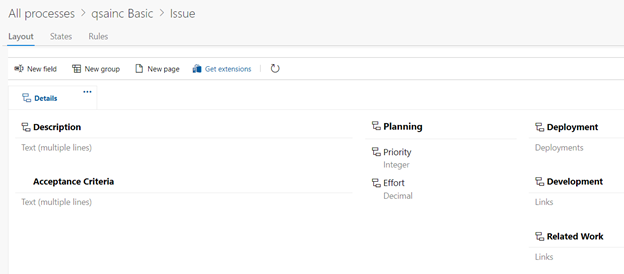
This field is added. Go back to your issue and you should see something like this;
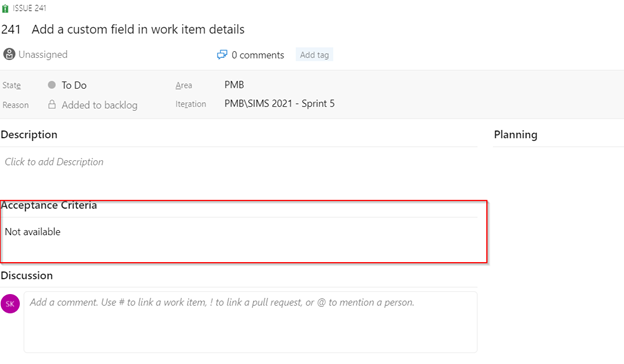
Hope this will save some time.