Knockout is a JavaScript library that implements MVVM pattern. It has a very small footprint and can be easily integrated in ASP.NET Core projects. It helps to solve complex logical UI challenges.
MVVM architecture is based on Martin Fowler’s Presentation Model. MVVM consists of three main components: Model, View, and ViewModel.
ViewModel references the Model where the View is completely unaware of the model. This helps front-end developer to stay focused on front-end development..
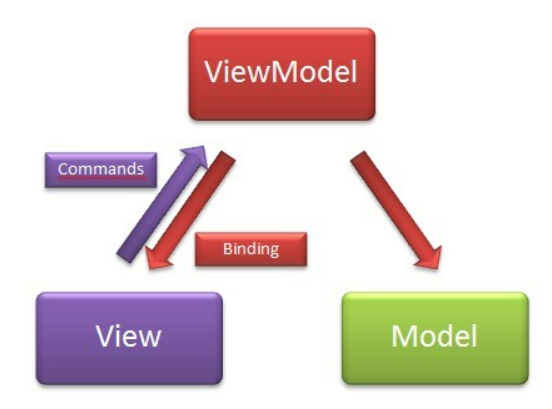
Here is a simple example using ASP.NET Core 6 MVC template.
<div>
The name is <span data-bind="text: personName"></span> and the age is <span data-bind="text: personAge"></span>
</div>
<div>
<span>PersonName: </span><input data-bind="value: personName" />
<span>PersonAge: </span><input data-bind="value: personAge" />
</div>
@section scripts {
<script type="text/javascript">
var myViewModel = {
personName: ko.observable('Shahzad'),
personAge: ko.observable(55)
};
//jQuery ready function
$(function () {
ko.applyBindings(myViewModel);
});
</script>
}
Some key functions from Knockout;
arrayFilter() -> Where()
arrayFirst() -> First()
arrayForEach() -> (no direct equivalent)
arrayGetDistictValues() -> Distinct()
arrayIndexOf() -> IndexOf()
arrayMap() -> Select()
arrayPushAll() -> (no direct equivalent)
arrayRemoveItem() -> (no direct equivalent)
compareArrays() -> (no direct equivalent)
For more info, refer here;
https://visualstudiomagazine.com/articles/2013/08/12/javascript-data-binding-with-knockout.aspx
https://blog.craigtp.co.uk/Post/2017/01/10/KnockoutJS_binding_on_SELECT_elements_in_a_ForEach_loop
Change event on select with knockout binding, how can I know if it is a real change?
Refresh Knockout bindings when viewModel changes
Interesting idea for dynamic queries

