I am receiving this dataset from a remote server;
TagNumber TagCode CageNumber FoodType FoodValue
A-2021-1 WHITE A9:I10 Milk A11:I
A-2021-1 WHITE A9:I10 Corn A10:I
A-2021-1 RED A11:I13 Meat B1:B2
A-2021-1 RED A11:I13 Hay A14:I
A-2021-1 GREEN A8:J9 Milk B1:B2
A-2021-1 GREEN A8:J9 Milk A10:J
I need to create this object, a complex type;
public class Animal
{
public string TagNumber { get; set; }
public string TagCode { get; set; }
public string CageNumber { get; set; }
public List<AnimalFood> Food { get; set; }
}
public class AnimalFood
{
public string FoodType { get; set; }
public string FoodValue { get; set; }
}
There could be many ways to handle this. This is one of them;
Create a dictionary object using LINQ GroupBy and TagCode column from incoming dataset;
var allAnimals = animalData
.GroupBy(item => item.TagCode)
.ToDictionary(grp => grp.Key, grp => grp.ToList());
Create unique animals out of dictionary object;
var uniqueAnimals = allAnimals.Keys.Distinct().ToList();
Create the object (animal) and add them to zoo :);
var zoo = new List<Animal>();
foreach (string animal in uniqueAnimals)
{
var animalRow = allAnimals[animal];
var animalRowFirst = animalRow.FirstOrDefault();
var animalRecord = new Animal
{
TagNumber = animalRowFirst.TagNumber,
TagCode = animalRowFirst.TagCode,
CageNumber = animalRowFirst.CageNumber
};
//time for food
var animalFood = new List<AnimalFood>();
foreach(var item in animalRow)
{
item.Food.ForEach(x =>
{
animalFood.Add(new AnimalFood
{
FoodType = x.FoodType,
FoodValue = x.FoodValue
});
});
}
//this is our custom type
animalRecord.Food = animalFood;
zoo.Add(animalRecord);
}
Sample output;
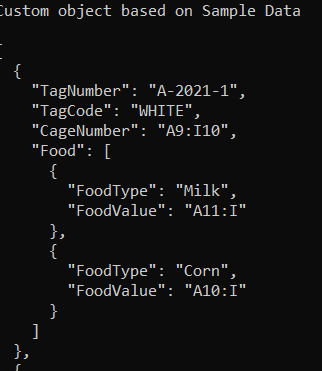
It’s easier to apply different filters on this object;
var animalFilter = zoo.Where(x => x.TagCode.ToUpper() == "WHITE");
Resources
https://stackoverflow.com/questions/3186818/unique-list-of-items-using-linq

