Let’s begin with a model.
public class RoleModel
{
public int RoleId { get; set; }
public string RoleName { get; set; }
}
Add some data to the model;
List<RoleModel> roles = new List<RoleModel> {
new RoleModel{
RoleId= 1,
RoleName = "Administrator"
},
new RoleModel
{
RoleId = 2,
RoleName = "Manager"
}
};
Pass this to razor view;
@model List<RoleModel>
Declare Javascript Sections in your razor view;
<end of HTML here>
@section Scripts
{
//go with jQuery ready function
$(document).ready(function () {
//get model data here
var roles = @Html.Raw(Json.Serialize(Model.Roles));
//iterate through model data
$.each(roles, function(i, role){
console.log(role.roleName);
});
}
}
This will output this data;
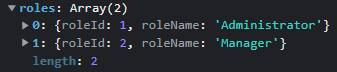
AspNetCore
AspNetCore uses Json.Serialize
intead of Json.Encode
var json = @Html.Raw(Json.Serialize(@Model.Roles));
MVC 5/6
You can use Newtonsoft for this:
@Html.Raw(Newtonsoft.Json.JsonConvert.SerializeObject(Model,
Newtonsoft.Json.Formatting.Indented))
This gives you more control of the json formatting i.e. indenting as above, camelcasing etc.

