I did a Web API deployment in Azure Web API service. I was able to access the URL in browser and Postman. I started getting following error when I try to integrate in ASP.NET Web App in my local development environment;
Access to XMLHttpRequest at ‘https://xyz-dev.azurewebsites.net/api/Authentication/GetTestUser?name=testuser’ from origin ‘http://localhost:17686’ has been blocked by CORS policy: Response to preflight request doesn’t pass access control check: No ‘Access-Control-Allow-Origin’ header is present on the requested resource.
jquery.js:9172 GET https://xyz-dev.azurewebsites.net/api/Authentication/GetTestUser?name=testuser net::ERR_FAILED
To solve the problem, I did this;
Here is server-side (Web API) code;
Add following to Web API ConfigureServices method;
public void ConfigureServices(IServiceCollection services)
{
services.AddCors(op =>
{
op.AddPolicy("AllOrigin", builder => builder.AllowAnyOrigin().AllowAnyMethod().AllowAnyHeader());
});
}
Add following to Web API Configure method;
public void Configure(IApplicationBuilder app, IWebHostEnvironment env, ILoggerFactory loggerFactory)
{
//-------use cords
app.UseCors("AllOrigin");
}
Here is client-side AJAX call.
<input type="button" id="btnWebApiCall" name=" btnWebApiCall" value="Test CORS" class=" btn btn-primary btn-lg justify-content-center" />
@section Scripts
{
<script>
$(document).ready(function () {
$("#btnAuthenticateSSO").click(function (e) {
e.preventDefault();
$.ajax({
type: "GET",
url: "https://xyzapi-dev.azurewebsites.net/api/Authentication/GetTestUser?name=testuser",
contentType: "application/json; charset=utf-8",
//crossDomain: true,
dataType: "json",
success: function (data, status, xhr) {
alert(JSON.stringify(data));
console.log(data);
}, //End of AJAX Success function
error: function (xhr, status, error) {
alert("Result: " + status + " " + error + " " + xhr.status + " " + xhr.statusText);
} //End of AJAX error function
});
});
});
</script>
}
The pain is gone.
If interested in learning more about this, read below;
Researched and figured out that browser sends two requests to the server. Tiny request and actual request.
The browser sends a tiny request called a preflight request before the actual request. It includes details such as the HTTP method used and whether any custom HTTP headers are present. The preflight allows the server to see how the actual request would appear before it is sent. The server will then tell the browser whether or not to submit the request, or whether to return an error to the client instead.
See below for problem header without CORS and with CORS in web API;
Headers without CORS implementation in Web API (Problem);

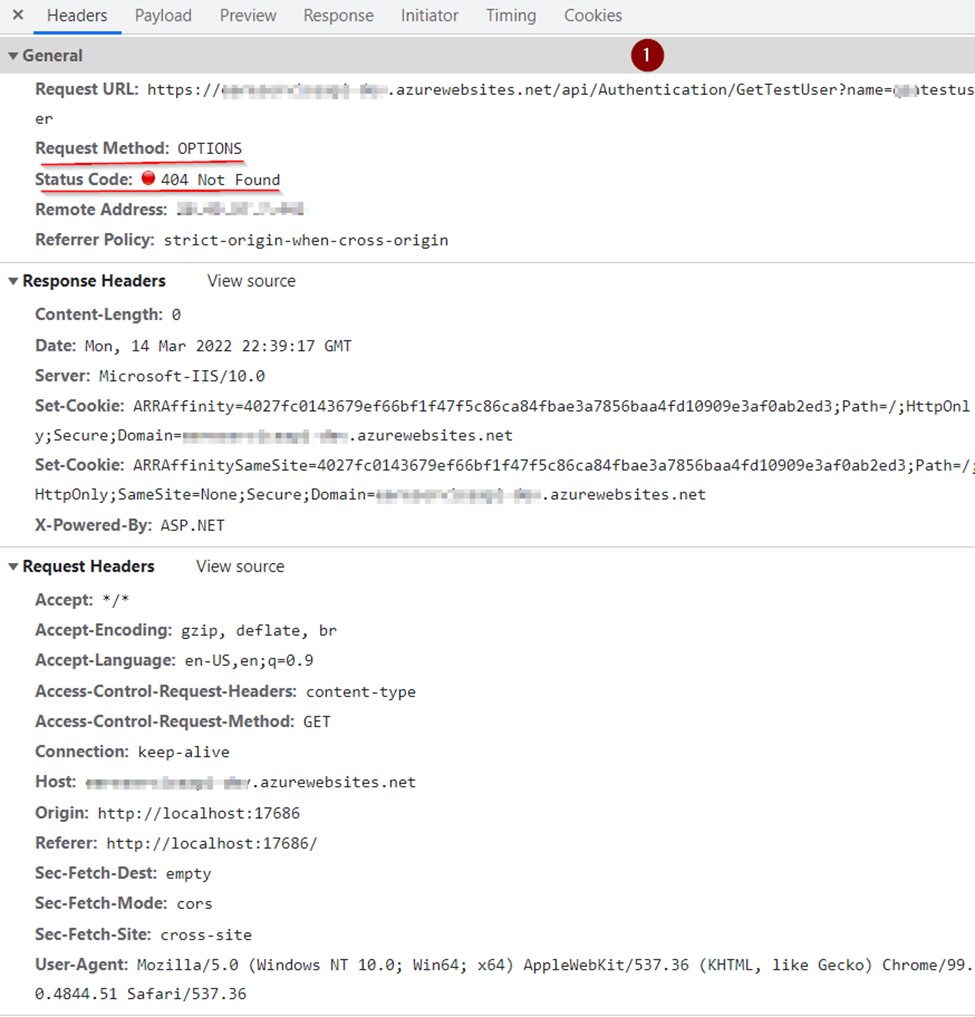
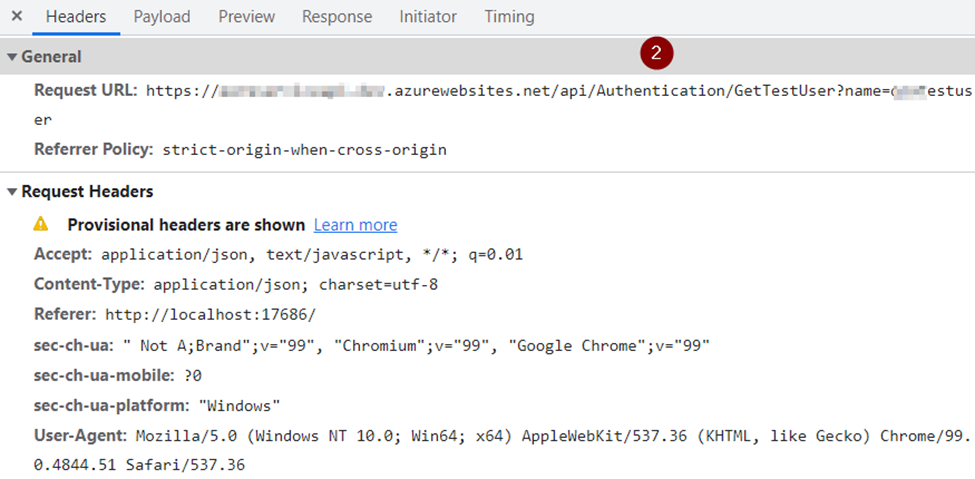
Headers with CORS implementation in Web API (Problem solved);
A simple Get request make these two requests;

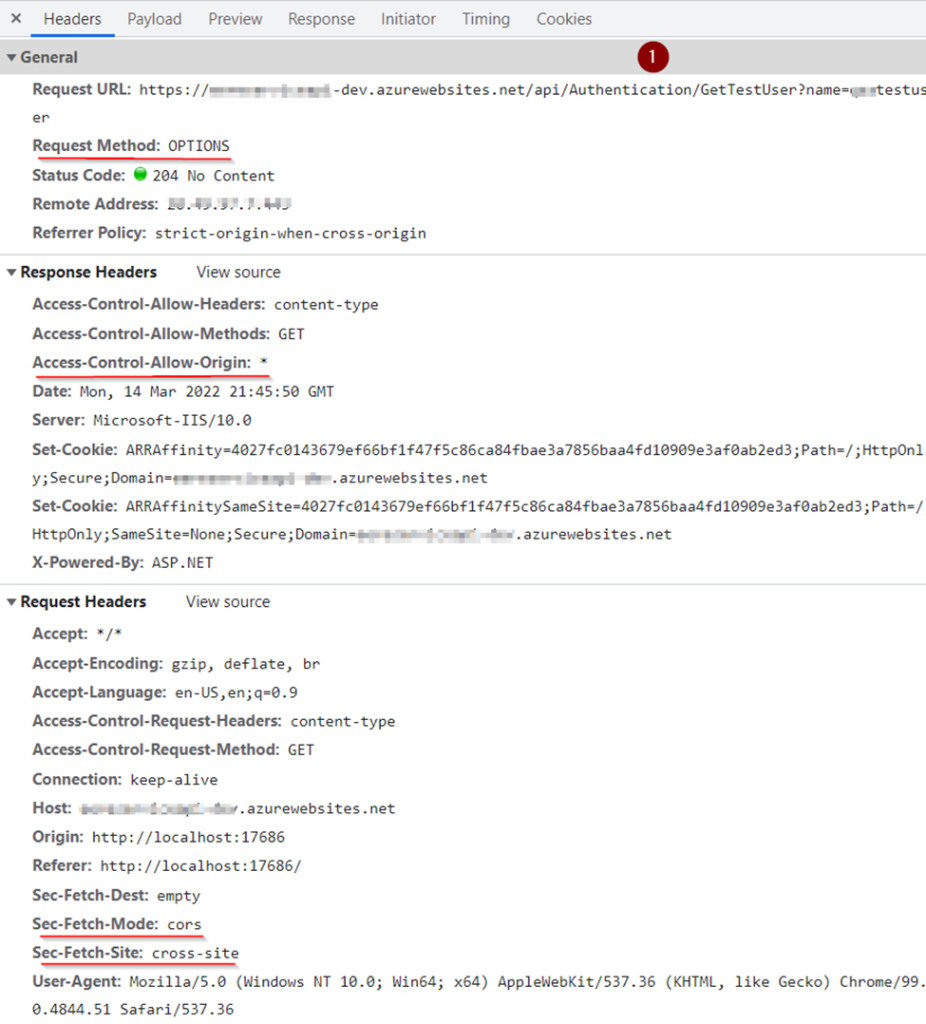
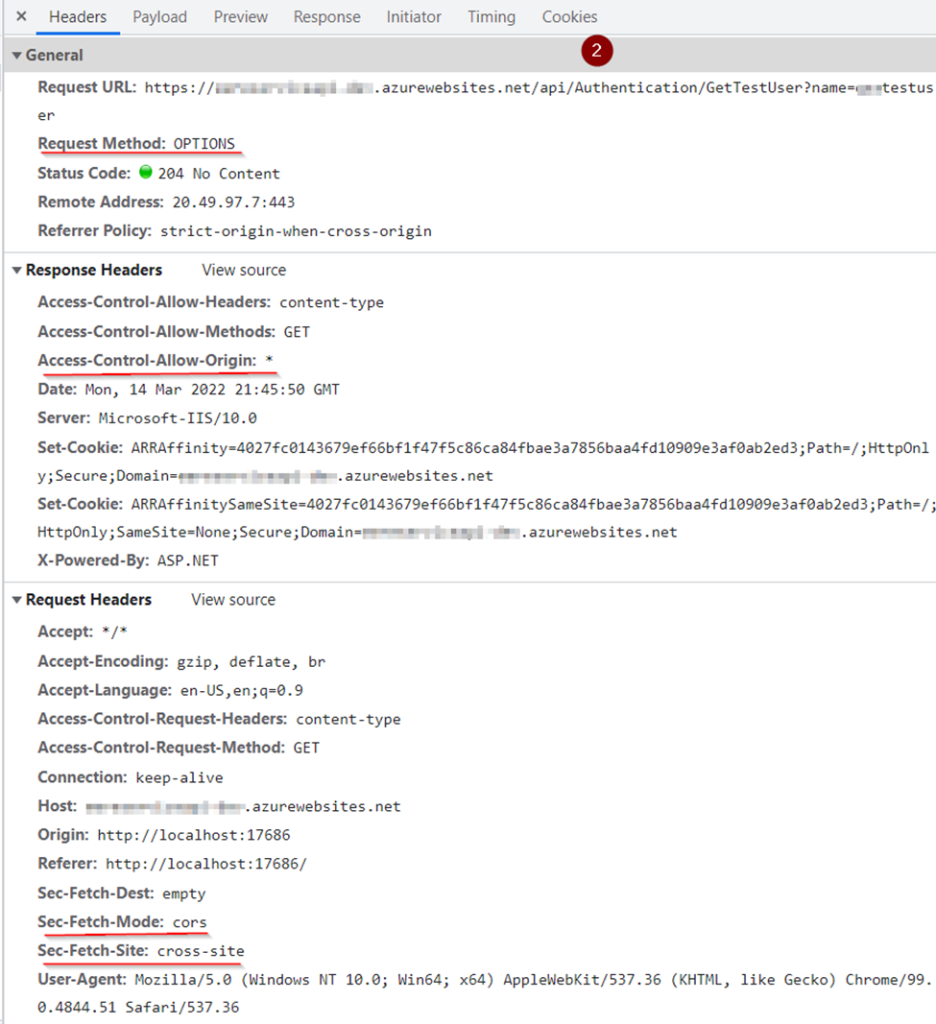
Resources
https://medium.com/easyread/enabling-cors-in-asp-net-web-api-4be930f97a5c
https://stackoverflow.com/questions/31942037/how-to-enable-cors-in-asp-net-core